十大经典排序算法 动图演示
https://www.cnblogs.com/onepixel/p/7674659.html
位运算
负数的二进制表示
十进制5在计算机中表示为 int类型占4个字节
00000000 00000000 00000000 00000101
那么-5在计算机中应该怎么表示呢?
在计算机中,负数以其正值的补码形式表达
什么叫补码呢?这得从原码,反码说起。
原码:一个整数,按照绝对值大小转换成的二进制数,称为原码。
比如 00000000 00000000 00000000 00000101 是 5的 原码。
反码:将二进制数按位取反,所得的新二进制数称为原二进制数的反码。
原码 00000000 00000000 00000000 00000101
反码 11111111 11111111 11111111 11111010
补码 11111111 11111111 11111111 11111011
补码: 反码+1称为补码
所以, -5在计算机中表达为:
11111111 11111111 11111111 11111011
转换为16进制: 0xFFFFFFFB
-1在计算机中如何表示
1的原码
00000000 00000000 00000000 00000001
1的反码
11111111 11111111 11111111 11111110
补码
11111111 11111111 11111111 11111111
可见, -1在计算机里用二进制表达就是全1
16进制为:0xFFFFFF
并查集
class UF {
// 记录连通分量
private int count
// 节点x的父节点是parent[x]
private int[] parent;
// 新增一个数组来记录树的“重量” 为了避免造成树的不平衡
private int[] size;
/*构造函数, n为图的节点总数*/
public UF(int n) {
// 一开始互不相通
this.count = n; // 连通分量的个数
// 父节点指针指向自己
parent = new int[n];
// 最初每棵树只有一个节点
// 重量应该初始化为1
size = new int[n];
for (int i=0; i<n; i++) {
parent[i] = i;
size[i] = 1; // 初始状态的节点个数为1
}
}
/* 将p和q连接 */
public void union(int p, int q) {
int rootP = find(p);
int rootQ = find(q);
if (rootP == rootQ)
return;
// 将两棵树合并为一颗
// 小树接到大树下面,较平衡
if (size[rootP] > size[rootQ]) {
parent[rootQ] = rootP;
size[rootP] += size[rootQ];
} else {
parent[rootP] = rootQ;
size[rootQ] += rootP;
}
count--; // 两个分量合二为一
}
/* 返回某个节点x的根节点 */
private int find(int x) {
// 根节点parent[x] == x
while (parent[x] != x) {
// 进行路径压缩
parent[x] = parent[parent[x]];
x = parent[x];
}
return x;
}
/* 判断p和q是否连通*/
public boolean connected(int p, int q) {
int rootP = find(p);
int rootQ = find(q);
return rootP == rootQ;
}
/* 返回图中有多少个联通分量 */
public int count() {
return count;
}
}
Minimax-Algorithm
—
title: Minimax Algorithm
date: 2020-08-14 20:03:12
tags:
categories: Algorithm
—
https://segmentfault.com/a/1190000013527949
const maxDepth = 4;
let counter = 1;
const dataTree = [
[
[
[3, 17], [2, 12]
],
[
[15], [25, 0]
]
],
[
[
[2, 5], [3]
]
[
[2, 14]
]
]
];
constructor(data, type, depth) {
this.data = data;
this.type = type; // 区分此节点的种类是max或min
this.depth = depth;
}
function changeType(type) {
return type === ‘max’ ? ‘min’ : ‘max’;
}
score() {
// 到达了最大深度后,此时的data是数组最内层的数字
if (this.depth >= 4) {
return this.data;
}
// 对于max节点,返回的是子节点中的最大值
if (this.type === 'max') {
let maxScore = -1000;
for (let i=0; i < this.data.length; i++) {
const d = this.data[i];
// 生成新的节点,子节点的type会和父节点不同
const childNode = new Node(d, changeType(this.type), this.depth+1);
// 递归获取其分数
const childScore = childNode.score();
if (childScore > maxScore) {
maxScore = childScore;
}
}
return maxScore;
}
// 对于min节点,返回的是子节点中的最小值
if (this.type === 'min') {
let minScore = 1000;
for (let i=0; i < this.data.length; i++) {
const d = this.data[i];
// 生成新的节点,子节点的type会和父节点不同
const childNode = new Node(d, changeType(this.type), this.depth+1);
// 递归获取其分数
const childScore = childNode.score();
if (childScore > minScore) {
minScore = childScore;
}
}
return minScore;
}
}
const testNode = new Node(dataTree, ‘max’, 0);
console.log(testNode.score());
Alpha-beta 剪枝算法的实现
是minimax的改进
class Node{
constructor(data, type, depth) {
this.data = data;
this.type = type; // 区分此节点的种类是max或min
this.depth = depth;
this.alpha = alpha || -Infinity;
this.beta = beta || Infinity;
}
score() {
}
}
AI
project1数独
约束传播和搜索
project2 Build an AI that play games
minimax alpha-beta pruning
吃豆人
BFS DFS A*
最优路径问题
project3 马尔可夫模型 手语字符转化为对应的英文文本
Navigation 导航 A*
智能体 动态交互
棋盘的状态 搜索树 剪枝
对抗搜索 Mini-Max
Monty Hall三门问题
switch
先验概率 P(A) = P(B) = P(C) = 1/3
后验概率 P(B|open_B) = 0
贝叶斯定理
P(C|open_B) = P(open_B|C) * P(C) / P(open_B) = 1 * 1/3 / 1/2 = 2/3
智能的定义是什么?
Agent, Enviroment, State
智能体:是指会根据给定目标采取行动,最大化其预期效用的事物
Perception, Action, Cognition
感知: 智能体通过感知环境属性与环境互动
行为:输出行为通常会改变环境的状态
认知:智能体决定采取什么行动的过程,取决于感知输入,这个过程称为认知
我们对AI的大部分讨论都会集中在认知上,特别是推理和决策
有限最优性
Conda与pip相似,不同之处在于可用的软件包集中于数据科学,而pip则用于一般用途。conda create -n env_name list of packages
conda create -n data python=3.6 numpy pandas
source activate env_name
conda list
查看安装的packagessource deactivate
保存和加载环境conda env export > enviroment.yaml
write the exported text to a YAML file
从保存的环境文件创建行动环境conda env create -f enviroment.yaml
create a new enviroment with the same listed in enviroment.yaml
移除环境conda env remove -n env_name
windows配置Git环境变量
https://blog.csdn.net/C_chuxin/article/details/87880332
搜索、逻辑、规划
概率、贝叶斯网络
Main topics:
- Adversarial Search
- Minimax Algorithm
- Alpha-Beta pruning
- Evaluation Functions
- Isolation Functions
- Multi-player, probabilistic Games
——————————————————————————————————————
路径搜索
Definition of A problem
Initial State -> S0 开始的城市
Actions(S) -> {a1, a2, a3…} 可到达的城市的路径
Result(S, a) -> S’ (到达)新的城市
GoalTest(S) -> True|False 判断是否到达目标城市
Path Cost(Si -aj-> Si+1 -aj+1-> Si+2)–> cost value(n) where i=0,1… j=1,2… 总的cost
Step Cost (S, a, S’) –> n 单步cost
Frontier 已访问的边界
Unexplored 未访问
Explored 已访问
Tree Search
function Tree.Search(problem):
frontier = {[initial]}
loop:
if frontier is empty:
return FAIL
path = remove.choice(frontier)
s = path.end
if s is a goal:
return path
for a in actions:
add[path + a > Result(s, a)] to frontier
Breadth First Search
shortest path first
Graph Search
function Graph.Search(problem):
frontier = {[initial]};
explored = {} // 记录已访问的节点
loop:
if frontier is empty:
return FAIL
path = remove.choice(frontier)
s = path.end; add to explored
if s is a goal:
return path
for a in actions:
add [path + a -> Result(s, a)] to frontier
unless Result(s, a) in frontier or explored // 避免重复
A*搜索算法
HTML & CSS
树形结构
h1元素
This is a heading.
This is a paragraph.
> 标签 This is a paragraph. 命令行启动sublime 添加完系统变量之后,接下来你就可以在命令行里面利用subl命令直接使用Sublime Text了: Chrome Paragraph of text. 前往 MDN HTML 元素参考 中文网站 ,寻找你要使用的元素。 HTML文档模版 按钮标签 标题标签
MAC 打开终端输入下面的命令并回车即可
ln -s /Applications/Sublime\ Text.app/Contents/SharedSupport/bin/subl /usr/local/bin/subl
subl file : 使用Sublime Text打开file文件
subl folder : 使用Sublime Text打开folder文件夹
subl . : 使用Sublime Text当前文件夹
cmd + o 打开文件
Cmd + R 刷新文件
递归嵌套Article Title
MDN (Mozilla Developer Network)
cmd + f 寻找关键字
bold 粗体
emphasis 着重
描述HTML的类型Hello!
HomePage
Welcome to my website
about me
more
SpaceX launched the Falcom Havey
列表标签
- HTML
- CSS
- JavaScript
HTML树
树形结构
你可以使用树形结构整理HTML文件,使它们更具有条理
这不仅有利于可读性,还与CSS、JavaScript的风格和编程形式保持了一致
使用链接元素创建超链接
谷歌
href代表“引用”
使用img元素创建图像 图像不需要结束标签
这里是一大段有关 Udacity 的文字。很酷!接下来是图像!
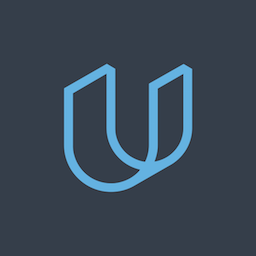
路径指南
绝对路径 :世界上任何人都可以使用地址 1600 Pennsylvania Ave NW, Washington DC, USA 20006 找到美国白宫。街道地址是指向某地点的绝对路径。
相对路径 :但如果你就在艾森豪行政大楼, 那么你还可以使用“隔壁大楼”来查找白宫。 “隔壁大楼”需要依赖当前位置,因此是一个相对路径。
本地文件
外部文件
本地路径
外部路径
相对路径
负责为你提供网站文件的计算机称为 服务器
服务器具有任何人都可以访问的外部路径,这也正是 Web 的工作原理。
不同的网站只不过是不同的文件集合。每个网站其实都只是具有外部地址的服务器(或多个服务器), 我们称外部地址为 URL
图片描述

斯托特纪念森林,杰迪戴亚·史密斯红木州立公园,2011 年,由 Chmee2 拍摄。GFDL 或 CC BY-SA 3.0,来自 Wikimedia Commons - source
UdacityDL
Anaconda
什么是Anaconda ?
Anaconda实际上是conda,Python和150多个科学软件包及其依赖项附带的软件发行版。应用程序conda是程序包和环境管理器。
安装Anaconda
Anaconda is available for Windows, Mac OS X, and Linux. You can find the installers and installation instructions at https://www.anaconda.com/download/.
安装后,您将自动进入默认的conda环境,并安装了所有软件包,您可以通过在终端中输入conda list
来检查自己的安装。
包管理
conda install numpy
创建环境
conda create -n env_name list of packages (-n for name)
conda create -n my_env numpy
指定python版本
conda create -n py38 python=3.8
进入环境
source activate my_env (OSX/Linux)
activate my_env (Windows)
conda create -n data py36 python=3.6 numpy pandas
保存和加载环境conda env export > enviroment.yaml
保存环境到yaml文件
conda env exportconda env create -f environment.yaml
从yaml文件创建环境
环境列表
conda env list
移除环境
conda env remove -n env_name